In .Net, during the compile time Metadata created with MSIL and stored it in a file called a Manifest . Both Metadata and Microsoft Intermediate Language together wrapped in a Portable Executable (PE) file and this can be accessed at runtime by a mechanism, called Reflection.
At runtime, the Reflection mechanism uses the Portable Executable file to read information about the assembly and it is possible to uncover the methods, properties, and events of a type, and to invoke them dynamically. Reflection generally begins with a call to a method present on every object in the .NET framework, GetType(). The GetType() is a member of the System.Object class, and the method returns an instance of System.Type.
The classes in the System.Reflection namespace, together with Type, enable we to get information about loaded assemblies and the types defined within them, such as classes, interfaces, and value types.
Q.What is .Net serialization?
Serialization can be defined as the process of converting the state of an object instance to a stream of data, so that it can be transported across the network or can be persisted in the storage location. The advantage of serialization is the ability to transmit data across the network in a cross-platform-compatible format, as well as saving it in a persistent or non-persistent state of an object to a storage medium so an exact copy can be recreated at a later stage. Deserialization is its reverse process, that is unpacking stream of bytes to their original form.
Any attempt to pass the object as a parameter or return it as a result will fail unless the object derives from MarshalByRefObject. This process of serializing an object is also called deflating or marshalling an object. If the object is marshalling or it is marked as Serializable, the object will automatically be serialized.
There are three types of serialization in .Net : Binary Serialization, SOAP Serialization and XML Serialization.
Binary Serialization
Binary serialization is the process where you convert your .NET objects into byte stream. In binary serialization all the public, private, even those which are read only, members are serialized and converted into bytes.
SOAP Serialization
SOAP is a protocol based on XML, designed specifically to transport procedure calls using XML. Because a SOAP message is built using XML, the XmlSerializer can be used to serialize classes and generate encoded SOAP messages.
XML Serialization
XML Serialization is the process of serializing a .Net Object to the form of XML or from an XML to .Net Object.
Q.Differences between Stack and Heap?
Stack and a Heap ?
Stack is used for static memory allocation and Heap for dynamic memory allocation, both stored in the computer's RAM .
Variables allocated on the stack are stored directly to the memory and access to this memory is very fast, and it's allocation is dealt with when the program is compiled. When a function or a method calls another function which in turns calls another function etc., the execution of all those functions remains suspended until the very last function returns its value. The stack is always reserved in a LIFO order, the most recently reserved block is always the next block to be freed. This makes it really simple to keep track of the stack, freeing a block from the stack is nothing more than adjusting one pointer.
Variables allocated on the heap have their memory allocated at run time and accessing this memory is a bit slower, but the heap size is only limited by the size of virtual memory . Element of the heap have no dependencies with each other and can always be accessed randomly at any time. You can allocate a block at any time and free it at any time. This makes it much more complex to keep track of which parts of the heap are allocated or free at any given time.
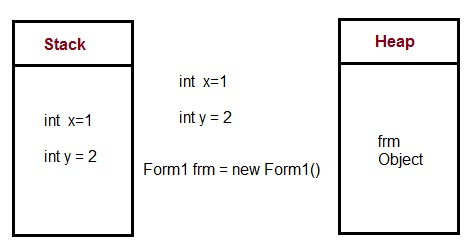
We can use the stack if we know exactly how much data you need to allocate before compile time and it is not too big. We can use heap if we don't know exactly how much data we will need at runtime or if we need to allocate a lot of data.
In a multi-threaded situation each thread will have its own completely independent stack but they will share the heap. Stack is thread specific and Heap is application specific. The stack is important to consider in exception handling and thread executions.
Q.Difference between a thread and a process?
Thread and a Process
The processes and threads are independent sequences of execution, the typical difference is that threads run in a shared memory space, while processes run in separate memory spaces.
A process has a self contained execution environment that means it has a complete, private set of basic run time resources particularly each process has its own memory space. Threads exist within a process and every process has at least one thread.
Each process provides the resources needed to execute a program. Each process is started with a single thread, known as the primary thread. A process can have multiple threads in addition to the primary thread.
On a multiprocessor system, multiple processes can be executed in parallel. Multiple threads of control can exploit the true parallelism possible on multiprocessor systems.
Threads have direct access to the data segment of its process but a processes have their own copy of the data segment of the parent process.
Changes to the main thread may affect the behavior of the other threads of the process while changes to the parent process does not affect child processes.
Processes are heavily dependent on system resources available while threads require minimal amounts of resource, so a process is considered as heavyweight while a thread is termed as a lightweight process.
What is multithreading ?
In .NET languages we can write applications that perform multiple tasks simultaneously. Tasks with the potential of holding up other tasks can execute on separate threads is known as multithreading.
No comments:
Post a Comment